Git Environment Setup
Installing Git
Adding SSH Key
- Create
ssh key
1$ ssh-keygen -t rsa -C "[email protected]"
- If cloning the repository fails, check the debug information
1$ ssh -vv -p 29418 [git server IP address]
- When verifying the publickey, the local machine provided the private key
/home/user/.ssh/id_rsa
, but there was no mutual signature algorithm. Attempts were made with algorithms like ed25529, but no matching authentication method was found. - The solution is to provide public and private key pairs for algorithms like ecdsa, ed25519, dsa, etc.
- Check the current OpenSSH version:
1$ ssh -V [git server IP address]

OpenSSH 8.8, considering
cryptographically broken
, has started to disable theRSA
signature algorithm using theSHA-1
hash algorithm. This is a client-side restriction, and we must provide key types that are recognized byOpenSSH 8.8
, such asEd25519
recommended byOpenSSH
.
- Using the Ed25519 algorithm
1# Generate ed25519 key
2$ ssh-keygen -t ed25519 -C "[email protected]"
3# Add the private key to the authentication agent
4$ ssh-add
Pulling a Remote Branch to Local
- Pull a remote branch to a specified local branch:
1$ git pull origin remote_branch_name:local_branch_name
- Pull a remote branch to the current local branch:
1$ git pull origin remote_branch_name
- Pull the remote branch with the same name as the current local branch to the current local branch (requires prior association with the remote branch):
1$ git pull
- Associate a local branch with a remote branch of the same name
1$ git push -u origin remote_branch_name
Pushing Local Changes to a Remote Branch
- Push the current local branch to a specified remote branch (note: pull is remote first then local, push is the opposite):
1$ git push origin local_branch_name:remote_branch_name
- Push the current local branch to a remote branch with the same name as the current local branch
1$ git push origin local_branch_name
- Push the current local branch to a remote branch with the same name as the current local branch (requires prior association with the remote branch)
1$ git push
Submitting Code via Gerrit
1$ git commit -m "xxx"
2$ git push origin HEAD:refs/for/master
Pay attention to
commit
submission standards
1$ git reset --soft HEAD^ # Undo the most recent commit
2$ git reset --soft HEAD^2 # Undo the last two commits
3$ git log # View recent commit logs
Amending a Commit
1$ git commit --amend # Amend the last commit
2$ git push origin HEAD:refs/for/master
Undoing a Mistaken git pull
git reflog
to view the history of changesgit reset --hard HEAD@{n}
(where n is the reference position you want to revert to), for example:1$ git reset --hard 911c4e90
If
git pull
is followed bygit reset --soft HEAD^
and it fails: Cannot do a soft reset in the middle of a merge1$ git reset --merge # Cancel the merge 2$ git rebase # Rebase the current branch
Resolving git pull
Conflicts (Deprecated)
Conflicts are scary. Now, the practice is to always pull before writing and before pushing.
Refer to: Resolving Conflicts During git pull
git push
(Deprecated)
In the past, it was common to git pull
and git push
without properly merging in order, which often led to push errors. The current approach is to create a new repository for each new feature, directly copying the latest code from gerrit and developing on that basis. This avoids many conflicts, and there is no need to frequently pull the latest code unless necessary, especially when dealing with existing conflicts. Carefully pull to local only after confirming the merge.
Modifying Commit Messages
git commit --amend
: Modify the message of the most recent commit.git rebase -i
: Modify the message of a specific commit.
Merging Multiple Commits
After committing once and pushing to remote, you made changes and committed again, then pushed. Later, the first commit was abandoned, and when merging, an error occurred because the first commit was missing, and the second commit contained modifications and additions. Locally, everything was fine, but colleagues encountered issues during the merge.
To merge these two commits, use the rebase command:
1$ git rebase -i HEAD~2
Change the second line from pick to squash or s (in order of commits). The editor here is not vim, but nano. To exit, press control + x
, then Y
to confirm, and press Enter to confirm the file name.
pick: execute this commit squash: this commit will be merged into the previous commit
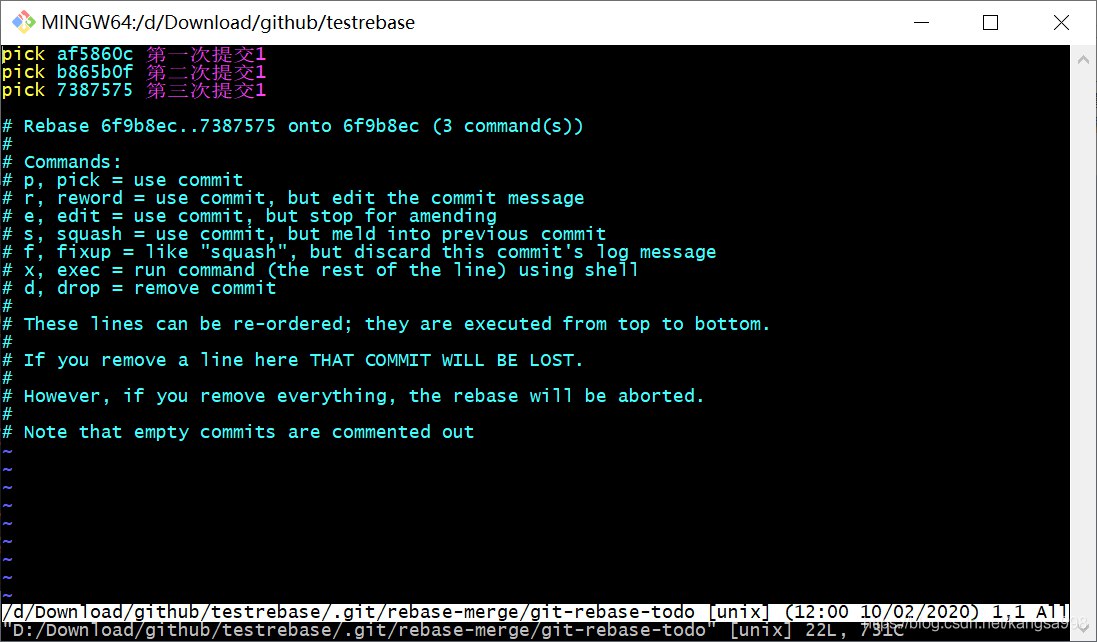
Comment out the unnecessary commit descriptions. I chose to comment out the old one because I needed to amend the change based on the previous one. After saving and exiting, there will be a successful prompt. After amending, push again.
git stash
1$ git stash save "message"
2$ git stash list # View existing stashes
3$ git stash pop # Reapply the most recent stash and delete it after applying
4$ git stash pop stash@{n} # Reapply a specific stash and delete it after applying
5$ git stash apply stash@{n} # Reapply a specific stash and keep it after applying
6
7$ git stash drop n # Remove a specific stash
8$ git stash clear # Delete all stashes
9
10$ git stash show stash@{1} # View the differences of a specific stash
git checkout
and git cherry-pick
To fetch unmerged code to local, there are generally two options (excluding direct modification and patching):
1$ git checkout FETCH_HEAD # The working directory reflects X
2$ git cherry-pick FETCH_HEAD # X fetches Y
In the first command, you simply switch to the remote changes, while in the last command, you apply the remote changes to the current branch.